Understand Decorator Design Pattern in Go with the help of a Coffee Shop Example | Go Code
Hey Folks, we are running a series of the design pattern available in the Go programming world. As of now we have discussed
We will understand the Decorator pattern using a simple coffee example. Lets assume we are building a coffee biller machine where there can be many top-ups available on the coffee.
Youtube Video on the same topic with wider coverage of topics available here: https://www.youtube.com/watch?v=_6-ivxilTZQ
Let’s assume you ordered a simple coffee
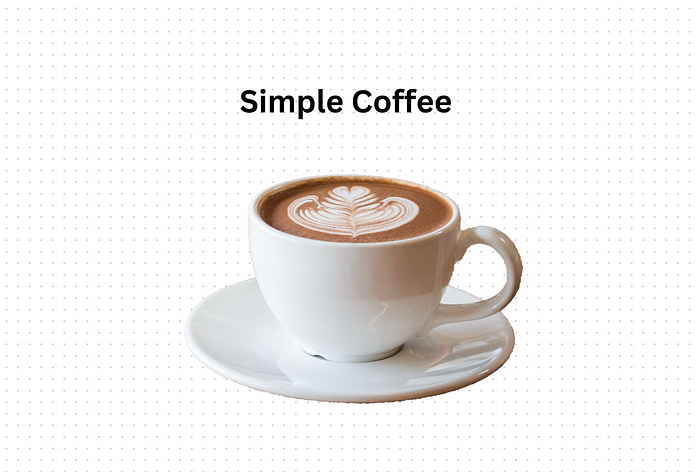
Coffee Bill have two fields which is description and cost, so lets define the interface for it first
// Coffee represents the base component interface
type Coffee interface {
GetDescription() string
Cost() float64
}
Let’s build this interface with our concrete component
// SimpleCoffee represents the concrete component
type SimpleCoffee struct{}
func (c *SimpleCoffee) GetDescription() string {
return "Simple Coffee"
}
func (c *SimpleCoffee) Cost() float64 {
return 1.0
}
Coffee maker: do you want to add extra milk?
You: Yes
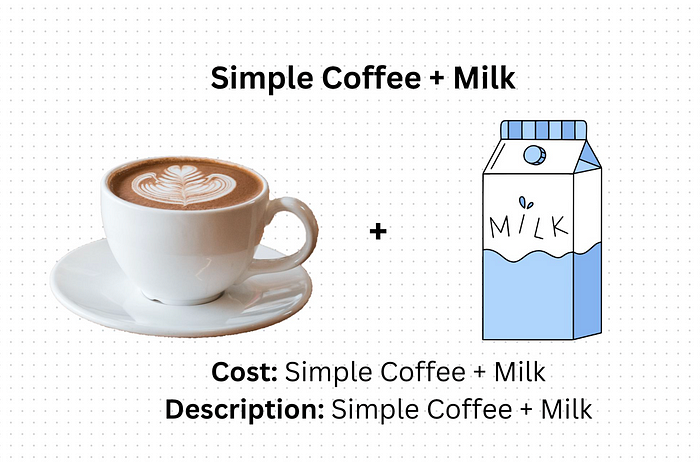
Let’s build the code for it too
// Milk represents a concrete decorator
type Milk struct {
*CoffeeDecorator
}
func NewMilk(coffee Coffee) *Milk {
return &Milk{&CoffeeDecorator{coffee}}
}
func (m *Milk) GetDescription() string {
return m.CoffeeDecorator.GetDescription() + ", Milk"
}
func (m *Milk) Cost() float64 {
return m.CoffeeDecorator.Cost() + 0.5
}
Coffee maker: Do you want extra sugar?
You: Yes

Let’s build the code for it too
// Sugar represents another concrete decorator
type Sugar struct {
*CoffeeDecorator
}
func NewSugar(coffee Coffee) *Sugar {
return &Sugar{&CoffeeDecorator{coffee}}
}
func (s *Sugar) GetDescription() string {
return s.CoffeeDecorator.GetDescription() + ", Sugar"
}
func (s *Sugar) Cost() float64 {
return s.CoffeeDecorator.Cost() + 0.25
}
Now let’s run the code and check the outout
func main() {
// Create a simple coffee
coffee := &SimpleCoffee{}
// Add milk to the coffee
coffeeWithMilk := NewMilk(coffee)
// Add sugar to the coffee
coffeeWithMilkAndSugar := NewSugar(coffeeWithMilk)
// Print description and cost of the decorated coffee
fmt.Println("Description:", coffeeWithMilkAndSugar.GetDescription())
fmt.Println("Cost:", coffeeWithMilkAndSugar.Cost())
}
Output:
Description: Simple Coffee, Milk, Sugar
Cost: 1.75
Full Code Github Link: CodePiper/GO/design-patterns/decorator.go at main · arshad404/CodePiper (github.com)
Youtube Video Link: https://www.youtube.com/watch?v=_6-ivxilTZQ
Stackademic 🎓
Thank you for reading until the end. Before you go:
- Please consider clapping and following the writer! 👏
- Follow us X | LinkedIn | YouTube | Discord
- Visit our other platforms: In Plain English | CoFeed | Venture | Cubed
- More content at Stackademic.com